This tutorial are talking about spring security base on servlet 3.1 with java-based configuration.
Before start,
- Using AbstractAnnotationConfigDispatcherServletInitializer to simplified web configuration. NO web.xml required.
- Spring platform to manage dependency
<properties> <java.version>1.8</java.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <io.spring.platform-version>1.1.2.RELEASE</io.spring.platform-version> </properties> <dependencyManagement> <dependencies> <dependency> <groupId>io.spring.platform</groupId> <artifactId>platform-bom</artifactId> <version>${io.spring.platform-version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <exclusions> <exclusion> <groupId>commons-logging</groupId> <artifactId>commons-logging</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-web</artifactId> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-config</artifactId> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-core</artifactId> </dependency> <!-- Refer to the source code ---> </dependencies>
Controller
Here we create 3 controller.
- IndexController has no added any security restriction
- IPSecureController restricted with ip address 127.0.0.1
- SecureController restricted with ROLE based login
@Controller public class IndexController { @RequestMapping("/") public String indexx() { return "index"; } } @Controller @RequestMapping("/ipsecure") public class IPSecureController { @RequestMapping(value = { "", "/", "/secretpage" } , method = RequestMethod.GET) public String indexx() { return "secureip"; } } @Controller @RequestMapping("/secure") public class SecureController { @RequestMapping(value = { "", "/", "/index"} , method = RequestMethod.GET) public String index() { return "securepage"; } }
Configuration
The following example extends WebSecurityConfigurerAdapter and configure path /secure required role admin to access and path /ipsecure to have ip address 127.0.01 therefore localhost will be prompt access denied.
@Configuration @EnableWebSecurity public class WebSecurityConfiguration extends WebSecurityConfigurerAdapter { @Autowired public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception { auth .inMemoryAuthentication() .withUser("user") .password("password") .roles("ADMIN"); } @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/secure/**").access("hasRole('ROLE_ADMIN')") .antMatchers("/ipsecure/**").access("hasIpAddress('127.0.0.1')") .and() .formLogin() .and() .httpBasic(); } }
WebAppInitializer
The important part here is to register WebSecurityConfiguration.class on getRootConfigurationClasses() method or either @import from the RootConfiguration class.
public class WebAppInitializer extends AbstractAnnotationConfigDispatcherServletInitializer { @Override protected Class<?>[] getRootConfigClasses() { return new Class<?>[] { RootConfiguration.class, WebSecurityConfiguration.class }; } @Override protected Class<?>[] getServletConfigClasses() { return new Class<?>[] { WebMvcConfiguration.class }; } @Override protected String[] getServletMappings() { return new String[] {"/"}; } }
SpringSecurityFilterChain
With web.xml configuration, we configure the spring security through filter and filter-mapping tags.
<filter> <filter-name>springSecurityFilterChain</filter-name> <filter-class> org.springframework.web.filter.DelegatingFilterProxy </filter-class> </filter> <filter-mapping> <filter-name>springSecurityFilterChain</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
AbstractSecurityWebApplicationInitializer
In java configuration, this is the all the code you need to register springSecurityFilterChain. This snippet of code is equivalent like above configuration. All you need to do is to create a class with extends AbstractSecurityWebApplicationInitializer.
public class WebSecurityInitializer extends AbstractSecurityWebApplicationInitializer { // intended leave blank }
How it works?
In short, AbstractSecurityWebApplicationInitializer class implements WebApplicationInitializer, and it execute a method insertSpringSecurityFilterChain to register springSecurityFilterChain during application bootstrapping.
Testing Scenario 1:
Start the server you’ll able see the page.
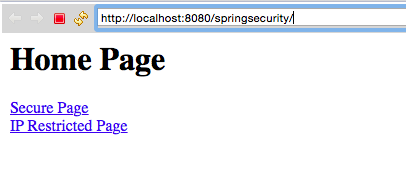
Testing Scenario 2:
Now click the Secure Page you’ll see the login form. Enter user and password click login. You’ll see the page below
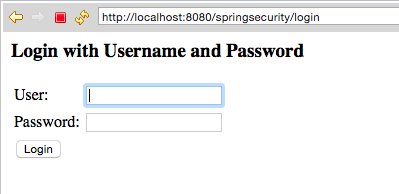

Testing Scenario 3:
Now click on the IP Restricted Page you will see access denied.
Change the URL from localhost to 127.0.0.1 you will see the page below
Source Code
https://github.com/loongest/springsecurity